2. Getting Started |
|
The only way to learn a new programming language is by writing programs in it. The first program to write is the "hello, world".
In C, the program to print "hello, world" is |
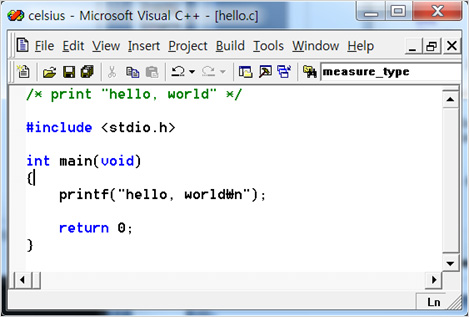 |
. |
2.1 Building a C Program
Compilation can involve up to four stages: preprocessing, compilation proper, assembly and linking, always in that order. The first three stages apply to an individual source file, and end by producing an object file; linking combines all the object files into an executable file.
The C compiler automatically compiles preprocessing, C compiling, and assembling at once. |
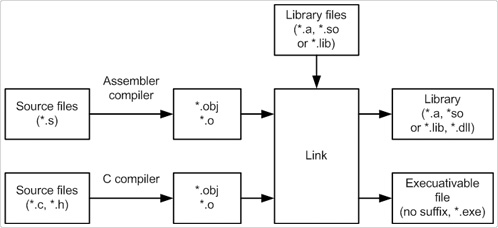 |
|
There are different files: |
Regular source code files.
|
– These files contain function definitions, and have names which end in ".c" by convention.
– Header files.
– These files contain function declarations (also known as function prototypes) and various preprocessor statements. They are used to allow source code files to access externally-defined functions. Header files end in ".h" by convention. |
Object files. |
– These files are produced as the output of the compiler. They consist of function definitions in binary form, but they are not executable by themselves. Object files end in ".o" by convention, although on some operating systems (e.g. Windows, MS-DOS), they often end in ".obj“. |
Library files. |
– Static library: A set of object files which are resolved in a caller at compile-time. The static libraries end in “.a” on Unix operating systems although they end in “.lib” on Windows.
– Dynamic library: The dynamic libraries end in “.so” on Unix operating systems, although they end in “.dll” on Windows. Dynamic libraries provide a mechanism for shared code and data, allowing a developer of shared code/data to upgrade functionality without requiring applications to be re-linked or re-compiled. |
Binary executables. |
– These are produced as the output of a program called a "linker". The linker links together a number of object files to produce a binary file which can be directly executed. Binary executables have no special suffix on Unix operating systems, although they generally end in ".exe" on Windows. |
|
2.2 Building a C Program on Windows |
|
2.2.1 Building in Visual Studio
You can build projects in Visual C++ in one of two ways: Using Visual Studio and Using the command line.
When building a Visual C++ application in Visual Studio, you can modify many of the build's settings in the project's Property Pages dialog box. |
|
STEP 1: [Build]-Compile [Ctrl-F7] |
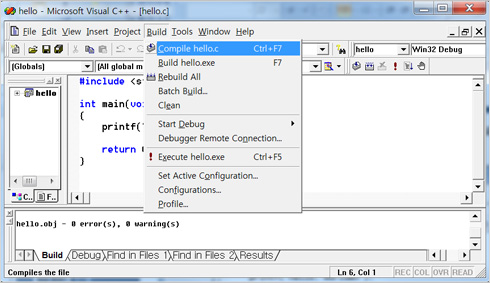 |
|
STEP 2: [Build]-Build [F7] |
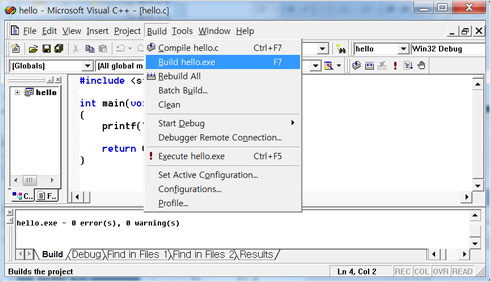 |
|
2.2.2 Building on the Command Line
Visual C++ provides command-line tools for programmers who prefer to build their applications from the command prompt. If you want to use the command line to build a project created in Visual C++, you can use one of the following: |
CL: Use the compiler (cl.exe) to compile and link source code files. |
Link: Use the linker (link.exe) to link compiled object files. |
MSBuild (Visual C++): Use MSBuild to build Visual C++ projects and Visual Studio solutions from the command line. Invoking this utility is equivalent to running the Build project or Build Solution command in the Visual Studio integrated development environment. |
DEVENV: Use DEVENV combined with a command line switch, such as /Build or /Clean, to perform certain build commands without displaying the Visual Studio IDE. |
NMake: Use NMake to automate tasks that build Visual C++ projects. |
|
Here, we will use the 'CL' command. The syntax is |
|
|
|
Options are specified by either a forward slash (/) or a dash (–). |
-c: Compile the source files, but do not link. By default, the object file name for a source file is made by replacing the suffix with `.o'. |
-o: Place output in file. If ‘-o' is not specified, the default is made by replacing the suffix with ‘.exe’ |
File is the name of one or more source files, .obj files, or libraries. |
|
An example is |
cl –c hello.c |
// hello.obj |
cl hello.c |
// hello.exe |
cl –o helloworld hello.c |
// helloworld.exe |
cl –o a.exe file1.c file2.c file3.obj |
|
|
|
|
To use CL command, first set the Path and Environment Variables for Command-Line Builds |
– Path |
Run vcvars32.bat by typing “vcvars32”. The vcvars32.bat file sets the appropriate environment variables to enable 32-bit command-line builds. |
Set the PATH to use “CL” command. |
Need mspdbXX.dll which is the Microsoft program database library used by Microsoft Visual Studio. |
– CL Environment Variables |
The CL tool processes options and arguments defined in the CL environment variable before processing the command line. |
|
SET CL=[ [option] ... [file] ...] [/link link-opt ...] |
|
|
vcvars32
path %PATH%;”C:\Program Files (x86)\Microsoft Visual Studio\VC98\Bin”
SET CL=/I”C:\Program Files (x86)\Microsoft Visual Studio\VC98\Include”
cl –o helloworld hello.c |
|
|
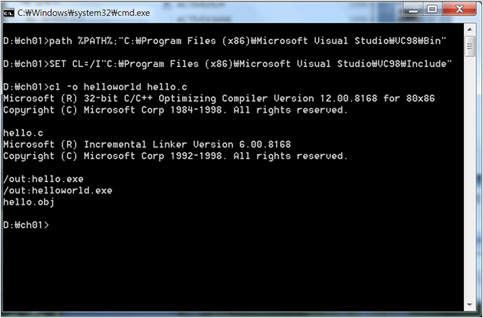 |
|
2.3 Building a C Program on Linux
By using "cc" or "gcc" compiler, we build a C program on Linux. The syntax is |
|
CC [option] filename |
// ‘gcc’ is identical to ‘cc’ |
|
|
|
Options are as follows: |
-c: Compile or assemble the source files, but do not link. The ultimate output is in the form of an object file for each source file. By default, the object file name for a source file is made by replacing the suffix `.c', `.i', `.s', etc., with `.o'. |
-o: Place output in file. If ‘-o' is not specified, the default is to put an executable file in ‘a.out’. |
-Wall: Enable all the warnings which the authors of cc believe are worthwhile. Despite the name, it will not enable all the warnings cc is capable of. |
-O: Create an optimized version of the executable. |
|
Example |
#cc hello.c |
// generate a.out |
#cc –o helloword hello.c |
|
#cc –c hello.c |
// generate hello.o |
#cc –o helloworld hello.o |
// generate helloworld |
#cc –O –o helloworld hello.c |
|
|
|
|
|
2.4 Building a C Program for a Embedded Linux
A cross compiler is a compiler capable of creating executable code for a platform other than the one on which the compiler is run. Figure 3 shows a process of the cross compile. |
|
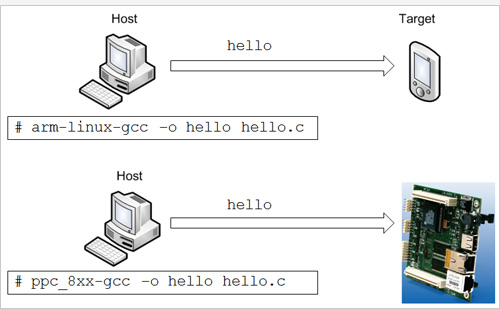 |
Fig. 3 An example of a cross compile |
|
|
2.5 Variables and Arithmetic Expressions |
|
Consider the following example. |
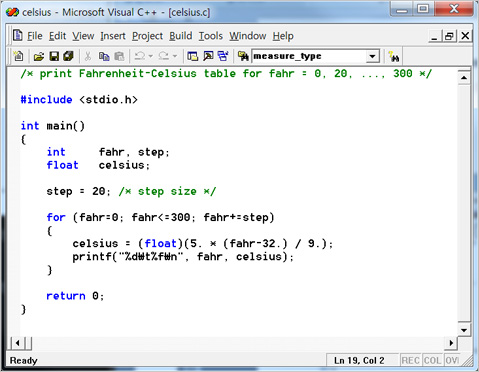 |
|
Variables |
– In ANSI C, all variables must be declared before they are used, usually at the beginning of the function before any executable statements.
– The size of these objects is also machine/compiler dependent. |
|
|
Table 2 summarizes the data type used in C. |
|
Table 2. Data type |
data type |
size
(Intel Windows 7 64 bits) |
description |
char |
1 |
character – a single byte |
short |
2 |
short integer |
int |
4 |
integer |
long |
4 |
long integer |
float |
4 |
float |
double |
8 |
double-precision float point |
|
|
Arithmetic operations are summarized as follows: |
C operation |
Arithmetic operator |
Addition |
+ |
Subtraction |
- |
Multiplication |
* |
Division |
/ |
Modulus |
% |
|
|
|
2.6 Input and Output
|
The standard output and input functions are printf() and scanf(). The conversion characters are |
Conversion character |
How the corresponding argument is printed or
How characters in the input stream are converted |
c |
as a character |
d |
as a decimal integer |
e |
as a floating-point number in scientific notitation |
f |
as a floating-point number (float) |
lf |
as a floating-point number (double) |
g |
in the e-format or f-format, whichever is shorter |
s |
as a string |
|
|