1. Functions
|
|
Functions break large computing tasks into smaller ones, and enable people to build on what others have done instead of starting over from scratch. Appropriate functions hide details of operation from parts of the program that don't need to know about them, thus clarifying the whole, and easing the pain of making changes. |
|
A structure of a source file ( .c) is as follows: |
/* Begin with comments about file contents */
Insert #include statements and preprocessor definitions
Function prototypes and variable declarations
Define main() function
{
Function body
}
Define other function
{
Function body
}
...
|
|
|
Developing a Large Program |
|
A large program consists of many source files and header files. |
We can place common materials in a header file, calc.h, which will be included as necessary. |
|
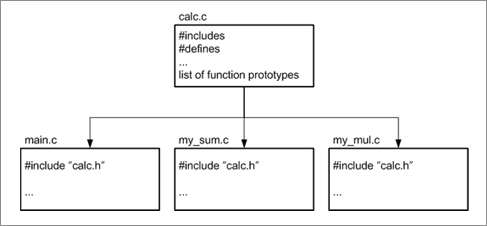 |
|
Compile |
– cc -o calc main.c myfunc1.c myfunc2.c |
Curly braces – combine into compound statement/block |
|
How to write a program using functions? |
|
Modular Programming |
– Conceptualize how a program can be broken into smaller parts
– C programs do not need to be monolithic
– Module: interface and implementation |
interface: header files |
implementation: auxiliary source/object files |
– Same concept carries over to external libraries |
|
Example: gcd program |
|
Compute the gcd(a, b), where get a and b from command line. |
Before coding |
– Break down problem into simpler sub-problems
– Consider iteration and recursion
– Minimize transfer of state between functions
– Writing pseudocode first can help |
/* The gcd() function */
int gcd(int a, int b)
{
while (b)
{
int temp = b;
b = a % b;
a = temp;
}
return a;
}
|
|
|
Make a Module |
|
Would like to include functionality in many programs |
Solution: make a module for the gcd(a, b) function |
Need to write header file (.h) and source file (.c) |
|
Example |
Header file
/* ensure included only once */
#ifndef __GCD_H__
#define __GCD_H__
/* compute gcd */
int gcd(int a, int b);
#endif
|
|