2. Creating a Static Library
|
|
file: libmath.h
#ifndef __LIBMATHFUNC_H__
#define __LIBMATHFUNC_H__
int plus(int a, int b);
int minus(int a, int b);
#endif
file: libmath.c
#include "libmath.h"
int plus(int a, int b)
{
return (a+b);
}
int minus(int a, int b)
{
return (a-b);
}
file: prog.c
#include <stdio.h>
#include "libmath.h"
int main()
{
printf("%d+%d=%d\n", 3, 2, plus(3, 2));
printf("%d+%d=%d\n", 3, 2, minus(3, 2));
return 0;
} |
|
|
2.1 Static Library on VC++ Command-Line |
|
Syntax |
suffix |
.lib |
command |
lib (Library Manager program) |
create |
cl /c /EHsc libmath.c
lib libmath.obj |
linking |
cl -o prog.exe prog.c libmath.lib |
|
|
Compiler options |
– /EH: Exception Handling
– /EHsc: catches C++ exceptions only and tells the compiler to assume that extern C functions never throw a C++ exception. |
|
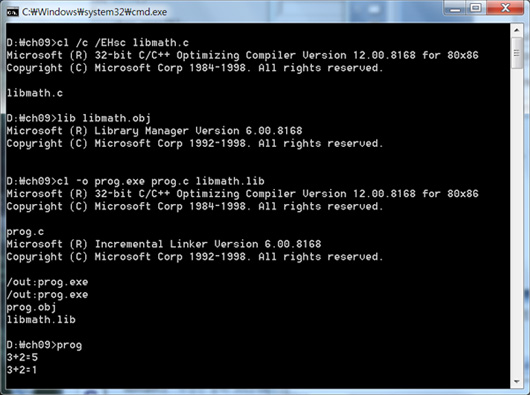 |
|
2.2 Static Library in Visual Studio |
|
Creating a new library project |
① From the File menu, select New and then Project.
② On the Project types pane, under Visual C++, select Win32.
③ On the Templates pane, select Win32 Console Application.
④ Choose a name for the project, such as libmath, and enter it in the Name field. Choose a name for the solution, such as StaticLibrary, and enter it in the Solution Name field.
⑤ Click OK to start the Win32 application wizard. On the Overview page of the Win32 Application Wizard dialog box, click Next.
⑥ On the Application Settings page of the Win32 Application Wizard, under Application type, select Static library.
⑦ On the Application Settings page of the Win32 Application Wizard, under Additional options, clear the Precompiled header check box.
⑧ Click Finish to create the project. |
To use the functionality from the static library in the application |
– Link a library module.. |
|
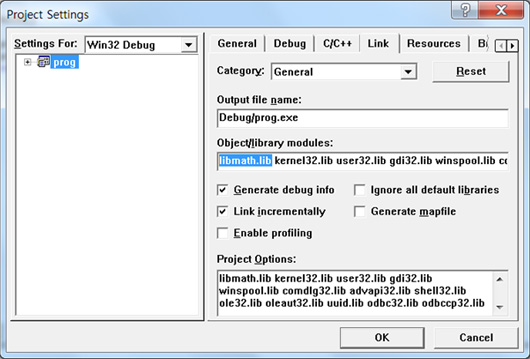 |
|
2.3 Static Library on Linux |
|
Syntax |
suffix |
.a |
command |
ar (archive program) |
create |
cc –Wall –c libmath.c
ar rc libmath.a libmath.o |
linking |
cc –o prog prog.c –L./ -lmath |
|
|
|
Compiler options |
– ar: create, modify, and extract from archives
|
r: Insert the files member into archive (with replacement).
c: Create the archive even if it did not exist. |
– cc |
-Wall: include warnings. See man page for warnings specified.
-llibrary: Search the library named library when linking. A file is named `liblibrary.a'
The directories searched include several standard system directories plus any that you specify
with `-L'.
|
|