|
3. Creating a Dynamic Link Library
|
|
3.1 DLL on VC++ Command-Line |
|
file: libmath2.h
#ifndef __LIBMATHFUNC_H__
#define __LIBMATHFUNC_H__
__declspec(dllexport) int plus(int a, int b);
__declspec(dllexport) int minus(int a, int b);
#endif
file: libmath2.c
#include "libmath.h“
__declspec(dllexport) int plus(int a, int b)
{
return (a+b);
}
__declspec(dllexport) int minus(int a, int b)
{
return (a-b);
} |
|
|
Note: “__declspec(dllexport)” modifiers enable the method to be exported by the DLL so that it can be used by other applications. |
|
Write and Compile the Implicit caller |
suffix |
.dll |
command |
cl |
create |
cl /LD libmath2.c [–o libmath2.dll] |
linking |
cl -o prog2.exe prog2.c libmath2.lib |
|
– /LD: creates a DLL. |
|
file: prog2.c
#include <stdio.h>
#include "libmath2.h"
int main()
{
printf("%d+%d=%d\n", 3, 2, plus(3, 2));
printf("%d+%d=%d\n", 3, 2, minus(3, 2));
return 0;
} |
|
|
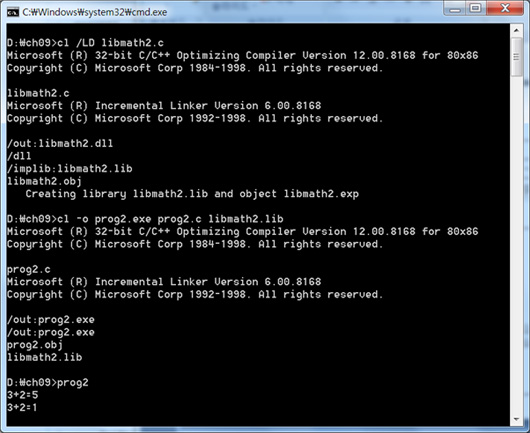 |
|
Write and Compile the explicit caller |
linking |
cl -o prog3.exe prog3.c |
|
|
#include <stdio.h>
#include <windows.h>
typedef int(*pPlus)(int, int);
typedef int(*pMinus)(int, int);
int main()
{
HINSTANCE hInstance;
pPlus plus;
pMinus minus;
if(!(hInstance=LoadLibrary("libmath2.dll")))
exit(1);
plus = (pPlus)GetProcAddress(hInstance, "plus");
minus = (pMinus)GetProcAddress(hInstance, "minus");
printf("%d+%d=%d\n", 3, 2, plus(3, 2));
printf("%d+%d=%d\n", 3, 2, minus(3, 2));
return 0;
} |
|
|
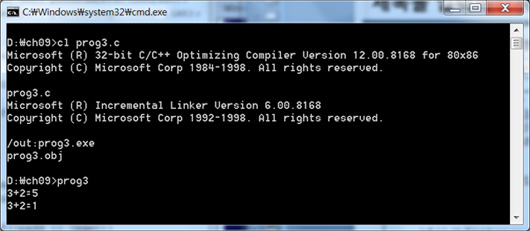 |
|
|
3.2 DLL in Visual Studio |
|
Creating a new DLL project in Visual Studio |
① From the File menu, select New and then Project….
② On the Project types pane, under Visual C++, select Win32.
③ On the Templates pane, select Win32 Console Application.
④ Choose a name for the project, such as libhello, and type it in the Name field. Choose a name for the solution, such as DynamicLibrary, and type it in the Solution Name field.
⑤ Click OK to start the Win32 application wizard. On the Overview page of the Win32 Application Wizard dialog box, click Next.
⑥ On the Application Settings page of the Win32 Application Wizard, under Application type, select DLL if it is available or Console application if DLL is not available. Some versions of Visual Studio do not support creating a DLL project by using wizards. You can change this later to make your project compile into a DLL.
⑦ On the Application Settings page of the Win32 Application Wizard, under Additional options, select Empty project.
⑧ Click Finish to create the project. |
|
The implicit caller |
– Link a library module.
|
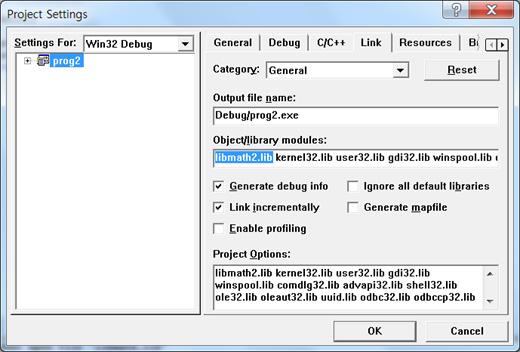 |
The explicit caller |
– Building without any setting.
|
|
|
|
|