2. Windows Multithreaded Programming
|
|
In C or C++ the program entry point is main or wmain (Unicode version). In windows application the program starts in WinMain or wWinMain. When the program starts, the operating system creates the first thread. Because, Windows is a multitasking operating system. |
|
Thread function |
|
Thread function is like an ordinary function with long void pointer parameter. We can pass any data through void pointer data type. |
– A simple thread function looks like |
|
void ThreadFunction(void *param)
{
// do somthing
} |
|
|
2.1 C Run Time Library Multithreading |
|
All the above c run time library functions are in the process.h header file. Make sure the Microsoft Visual Studio project setting is as multithreaded DLL. |
|
Function |
Description |
create threads |
_beginthread |
use _endthread |
_beginthreadex |
some addition parameters like security and thread address. |
end the thread |
_endthread |
It closes thread handle automatically. |
_endthreadex |
We closes the thread handle using CloseHandle Win32 API function. |
|
– Note: Microsoft recommend that If you use _beginthread functions for C run Time Library you can not use Win32 API like ExitThread or CreateThread. Because, if use that method it might result in deadlocks. |
|
unsigned long _beginthread(
void( __cdecl *start_address )( void * ),
unsigned stack_size,
void *arglist );
void _endthread( void ); |
|
|
start_address: Start address of routine that begins execution of new thread
stack_size: Stack size for new thread or 0
arglist: Argument list to be passed to new thread or NULL
If successful, each of these functions returns a handle to the newly created thread. _beginthread returns –1 on an error |
|
Project Settings: Make sure the Microsoft Visual Studio project setting is as multithreaded DLL. |
|
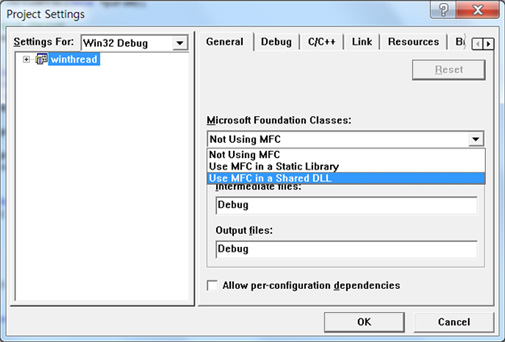 |
|
Example |
|
#include <stdio.h>
#include <windows.h>
#include <process.h> /* _beginthread, _endthread */
void ThreadProc(void *param)
{
int h=*((int*)param);
printf("%d Thread is Running!\n", h);
_endthread();
}
int main()
{
int n, i; int val = 0;
HANDLE handle;
printf("Enter the number of threads : ");
scanf("%d",&n);
for(i=0; i<n; i++)
{
val = i;
handle = (HANDLE) _beginthread(ThreadProc, 0, &val);
WaitForSingleObject(handle, INFINITE);
}
return 0;
} |
|
|
2.2 MFC Multithreaded |
|
The CWinThread is the base class for all thread operations. |
– The MFC class hierarchy
– CObject::CCmdTarget::CWinThread::CWinApp |
Data members |
– m_hThread The current thread handle
– m_bAutoDelete Whether the thread has set to auto delete or not
– m_nThreadID The current thread ID |
Data Functions: |
– CreateThread Start the exec execution of thread
– SuspendThread Increment thread suspend count. When the resume thread occur only the thread resume.
– ResumeThread Resume the thread. Decrements the thread count stack
– SetThreadPriority Set thread priority ( LOW, BELOW LOW or HIGH)
– GetThreadPriority Get the thread Priority |
|
Not all the member functions in MFC as class members. We can access some functions globally also. These functions begin with Afx.
|
|
CWinThread* AfxBeginThread(
AFX_THREADPROC ThreadProc,
LPVOID Param,
int nPriority = THREAD_PRIORITY_NORMAL,
UINT nStackSize = 0,
DWORD dwCreateFlags = 0,
LPSECURITY_ATTRIBUTES lpSecurityAttrs = NULL );
void AfxEndThread( UINT nExitCode ); |
|
|
Example |
CwinThread *pThread = AfxBeginThread( ThreadFunction, &data);
UINT ThreadFunction(LPVOID param)
{
DWORD result =0 ;
// do somethig
AfxEndThread(exitCode);
return result;
} |
|
|
2.3 Win32 Multithreaded |
|
Win32 Thread created by CreateThread function. The syntax in CreateThread is following |
HANDLE CreateThread(
LPSECURITY_ATTRIBUTES lpThreadAttributes,
DWORD dwStackSize,
LPTHREAD_START_ROUTINE lpStartAddress,
LPVOID lpParameter,
DWORD dwCreationFlags,
LPDWORD lpThreadId); |
|
|
We terminate thread using the following methods. |
– 1. use TerminateThread function
– 2. use ExitThread function
– 3. use return
– But, Advanced Windows by Jeffery Ritcher recommends us to use the return method. |
|
|